Top Data Structures Every Programmer Should Know
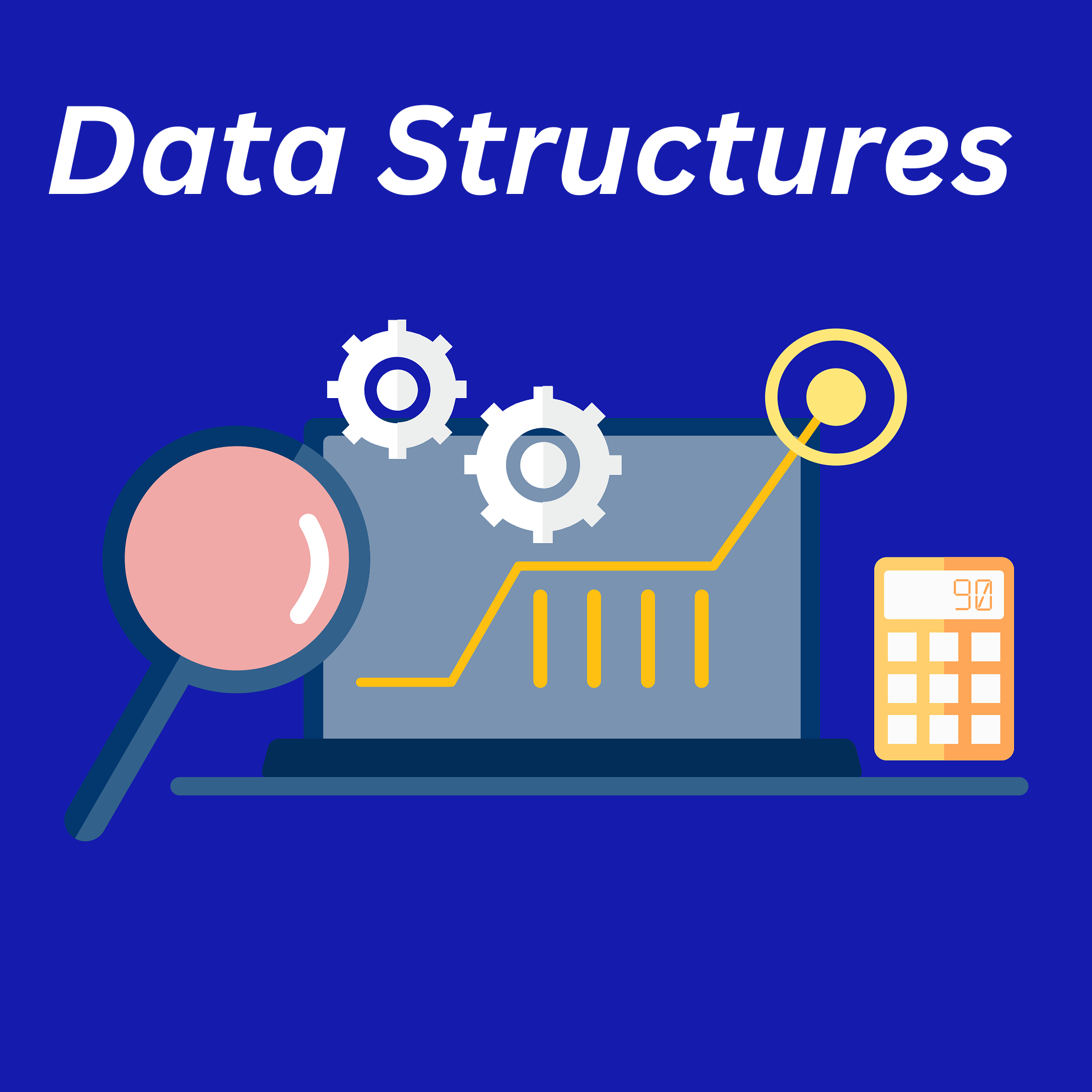
Introduction to Data Structures and Algorithms
Data structures and algorithms are fundamental concepts in computer programming. Data structures refer to the way data is organized and stored in a computer, while algorithms are step-by-step procedures for solving problems and performing tasks. Understanding data structures is essential for writing efficient and scalable code.
Importance of Data Structures and Algorithms
- Efficiency: Using the right data structure and algorithm can significantly improve the performance of a program.
- Scalability: Well-designed data structures can handle large amounts of data without slowing down.
- Reusability: Understanding different data structures allows programmers to reuse code in various projects.
- Problem-solving: Algorithms provide systematic approaches to solving complex problems efficiently.
Common Data Structures
- Arrays: A collection of elements stored in contiguous memory locations.
- Linked Lists: Each element points to the next, forming a sequence.
- Stacks: Follows the Last In, First Out (LIFO) principle.
- Queues: Follows the First In, First Out (FIFO) principle.
- Trees: Hierarchical data structures with a root node and child nodes.
- Graphs: Represented by nodes (vertices) connected by edges.
Basic Algorithms
- Search Algorithms: Finding a specific value in a collection of data.
- Sorting Algorithms: Arranging elements in a specific order, e.g., ascending or descending.
- Recursive Algorithms: Functions that call themselves to solve subproblems.
- Greedy Algorithms: Making the best choice at each step to find an optimal solution.
Understanding these data structures and algorithms is crucial for any programmer looking to write efficient and scalable code. The following sections will delve deeper into each data structure, providing insights into their implementation and use cases.
The Importance of Data Structures and Algorithms in Software Development
-
Data structures and algorithms form the foundation of software development and are essential for writing efficient and optimized code.
-
Using the right data structure can significantly impact the performance of an application, as it determines how data is stored, accessed, and manipulated.
-
Efficient algorithms are crucial for solving complex problems and improving the overall speed and scalability of software applications.
-
Understanding data structures and algorithms enables programmers to write code that is more organized, readable, and maintainable.
-
Data structures like arrays, linked lists, stacks, queues, trees, and graphs are commonly used in various programming scenarios to store and manipulate data efficiently.
-
Algorithms such as sorting, searching, and traversal play a vital role in optimizing the performance of software applications.
-
Proficiency in data structures and algorithms is often a key requirement for tech interviews and job opportunities in the software development field.
-
Continuous learning and practice of data structures and algorithms can enhance a programmer's problem-solving skills and ability to write efficient code.
-
Overall, a solid understanding of data structures and algorithms is crucial for any programmer looking to excel in software development and build high-quality applications.
Common Data Structures and Their Impact on Software Development
-
Lists (Array, Linked List):
- Arrays are essential data structures that store elements of the same type sequentially in memory, allowing for fast access. Linked lists provide dynamic memory allocation for elements and efficient insertions and deletions but have slower access times compared to arrays.
-
Stacks:
- Stacks operate on a Last In, First Out (LIFO) principle. They are crucial for functions like undo mechanisms and parsing expressions. Implementing a stack effectively can enhance the efficiency of algorithms.
-
Queues:
- Queues follow a First In, First Out (FIFO) approach. They play a significant role in managing tasks that need to be processed in order. Priority queues extend this concept by assigning a priority to each element.
-
Trees (Binary Tree, Binary Search Tree, AVL Tree):
- Trees are hierarchical data structures with a root node and child nodes. Binary trees have at most two children per node, while binary search trees maintain order for efficient search operations. AVL trees are self-balancing, ensuring logarithmic time complexity for common operations.
-
Hash Tables:
- Hash tables offer constant-time average case complexity for insertions, deletions, and lookups. They provide efficient retrieval based on a key, making them valuable for applications like caching and indexing.
-
Graphs:
- Graphs represent relationships between data points. They are crucial in social networks, map applications, and routing algorithms. Implementing efficient graph traversal algorithms can significantly impact performance.
Understanding these common data structures and their characteristics is paramount for software developers. Choosing the right data structure can lead to optimized algorithms, reduced time complexity, and improved performance of software applications.
Algorithms and Their Role in Software Development
Algorithms play a crucial role in software development, as they are the step-by-step procedures that outline how to perform a task or solve a problem. They are essential for manipulating data structures efficiently and accurately. Understanding algorithms is fundamental for programmers to write code that is both efficient and effective.
- Algorithms are the cornerstone of computer programming. They provide a clear set of instructions to solve specific problems or perform certain tasks.
- When developing software, programmers use algorithms to manipulate data structures. This manipulation is key to organizing and utilizing data effectively within a program.
- By implementing efficient algorithms, developers can ensure that their software runs smoothly and performs tasks in an optimal manner.
- Understanding different algorithms allows programmers to select the most appropriate one for a particular task, based on factors like time complexity and space complexity.
- Along with data structures, algorithms form the backbone of software development. They enable developers to create solutions that are scalable, adaptable, and efficient.
- As technology advances and the demand for cutting-edge software grows, the ability to design and implement efficient algorithms becomes increasingly valuable in the programming world.
In conclusion, mastering algorithms is essential for every programmer. They not only help in solving problems but also contribute to the overall efficiency and performance of software applications.
Performance Optimization through Data Structures and Algorithms
-
Data Structures:
- Efficient data structures are crucial for optimizing the performance of algorithms in programming.
- Arrays provide fast access to elements based on their index, making them ideal for operations like search and retrieval.
- Linked Lists offer dynamic memory allocation and efficient insertion and deletion of elements at any position.
- Stacks and Queues are essential for managing data in a Last-In-First-Out (LIFO) and First-In-First-Out (FIFO) manner, respectively.
- Trees, such as Binary Search Trees and AVL Trees, are useful for organizing data hierarchically for quick search and traversal.
- Graphs enable the representation of complex relationships between data points, essential for applications like social networks and mapping.
- Heaps are efficient for maintaining priority queues and sorting algorithms like Heapsort.
-
Algorithms:
- Sorting algorithms like Bubble Sort, Quick Sort, and Merge Sort are fundamental for arranging data in a specific order for efficient retrieval.
- Searching algorithms such as Linear Search and Binary Search aid in finding elements in a dataset quickly.
- Graph algorithms like Depth-First Search (DFS) and Breadth-First Search (BFS) assist in exploring and traversing graph structures.
- Dynamic Programming is beneficial for solving complex problems by breaking them down into simpler subproblems and reusing solutions.
- Greedy Algorithms make decisions at each step to reach an optimal solution, suitable for optimization problems.
By mastering these data structures and algorithms, programmers can significantly enhance the performance of their code by efficiently storing, retrieving, and manipulating data. This optimization leads to faster execution times, reduced resource consumption, and overall improved software efficiency.
Data Structures and Algorithms in Different Programming Languages
- Data structures and algorithms are fundamental concepts in programming that every programmer should be well-versed in.
- Understanding how data is organized and manipulated, as well as the efficiency of algorithms, is crucial for writing efficient and optimized code.
Common Data Structures:
- Arrays
- Linked Lists
- Stacks
- Queues
- Trees
- Graphs
- Hash Tables
These data structures are commonly used in various programming languages such as:
- C++
- Java
- Python
- JavaScript
- C#
- Ruby
- Go
- Swift
Each programming language has its own way of implementing and utilizing data structures and algorithms. For example, C++ provides built-in support for standard template library (STL), which offers pre-implemented data structures like vectors, queues, and maps. Java has a rich collection framework providing classes like ArrayList, LinkedList, and HashMap for efficient data management. Python offers versatile data structures like lists, tuples, dictionaries, and sets, making it a popular choice for beginners and professionals alike.
Here is a brief overview of implementation examples across some common programming languages:
C++:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> v = {1, 2, 3, 4, 5};
for (int i = 0; i < v.size(); ++i) {
cout << v[i] << " ";
}
return 0;
}
Java:
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList<Integer> list = new ArrayList<>();
list.add(1);
list.add(2);
list.add(3);
for (Integer num : list) {
System.out.print(num + " ");
}
}
}
Python:
my_list = [1, 2, 3, 4, 5]
for num in my_list:
print(num, end=" ")
Understanding data structures and algorithms in different programming languages allows programmers to choose the most suitable approach for solving specific problems efficiently and effectively.
Real-world Applications of Data Structures and Algorithms
- Data structures like arrays are commonly used in real-world applications such as managing contact lists, databases, and handling large datasets efficiently.
- Linked lists find applications in image viewer apps where users can navigate through a playlist or a set of images.
- Stacks are widely used in undo mechanisms in text editors, backtracking in algorithms, browser history management, and function call management in programming languages.
- Queues play a crucial role in job scheduling in operating systems, printing tasks in networks, and simulating scenarios in various applications.
- Trees are utilized in file systems to organize files and folders, in artificial intelligence for decision-making processes, and in HTML parsing to represent the structure of web pages.
- Graphs are fundamental in social networks to represent connections between users, in GPS systems for finding the shortest route, and in recommendation systems to suggest relevant items.
Data structures and algorithms are the backbone of computer science and are essential components in software development. Understanding their real-world applications helps programmers choose the right data structures to optimize performance and solve complex problems efficiently.
Challenges in Implementing Data Structures and Algorithms in Software Development
- Implementing complex data structures and algorithms can be challenging for programmers, especially when dealing with large-scale systems.
- Choosing the appropriate data structure for a specific problem can be difficult and requires a deep understanding of the characteristics and behavior of each structure.
- Ensuring the efficiency and scalability of data structures and algorithms in real-world applications can be a major challenge, as performance bottlenecks may arise.
- Debugging and optimizing data structures and algorithms in code can be time-consuming, requiring careful analysis and testing to identify and resolve issues.
- Adapting existing data structures and algorithms to meet the unique requirements of a particular software project can be a complex task that demands creative problem-solving skills.
- Keeping up-to-date with new data structures and algorithms in the ever-evolving field of computer science can be challenging, as programmers must continually learn and adapt to new techniques.
- Balancing the trade-offs between time complexity, space complexity, and ease of implementation when selecting data structures and algorithms can pose a significant challenge for developers.
- Collaborating with team members to implement data structures and algorithms in a cohesive and consistent manner across a project can be challenging, requiring effective communication and coordination.
These challenges highlight the importance of continuous learning, practice, and collaboration in mastering the implementation of data structures and algorithms in software development.
The Future of Data Structures and Algorithms in Software Development
The future of data structures and algorithms in software development is promising, with ongoing advancements in technology and the increasing demand for high-performing applications. Here are some key points to consider:
-
Increased Complexity: As software systems become more complex, the need for efficient data structures and algorithms will continue to grow. Developing innovative solutions to handle vast amounts of data efficiently will be crucial for the success of future applications.
-
Artificial Intelligence and Machine Learning: With the rise of AI and machine learning technologies, the importance of optimized algorithms and data structures cannot be overstated. These technologies heavily rely on sophisticated algorithms to process and analyze data accurately and quickly.
-
Big Data: The era of big data presents unique challenges for developers. Implementing data structures that can handle massive datasets in real-time is essential. Algorithms that can efficiently process, analyze, and extract valuable insights from big data will be in high demand.
-
Internet of Things (IoT): IoT devices generate a vast amount of data that needs to be collected, processed, and analyzed in real-time. Developing data structures and algorithms optimized for IoT applications will be crucial for ensuring the seamless operation of interconnected devices.
-
Quantum Computing: The emergence of quantum computing poses new challenges and opportunities for data structures and algorithms. Developers will need to explore quantum data structures and algorithms to harness the power of quantum computers efficiently.
In conclusion, staying up-to-date with the latest advancements in data structures and algorithms will be paramount for software developers looking to build scalable, efficient, and innovative applications in the future. Embracing new technologies and mastering fundamental data structures will be essential for success in the ever-evolving field of software development.
Learn Data Structures And Algorithms
Conclusion
- Data structures are the building blocks of programming, allowing developers to store and organize data efficiently.
- Understanding different data structures can help programmers select the most suitable one for each task.
- Arrays and linked lists are fundamental data structures that every programmer should be familiar with.
- Stacks and queues are essential for managing data in a last-in, first-out or first-in, first-out manner.
- Trees and graphs are powerful data structures for representing hierarchical and non-linear relationships.
- Hash tables provide fast access to data through key-value pairs, making them efficient for lookups and insertions.
- Understanding the strengths and weaknesses of each data structure is crucial for writing efficient and optimized code.
- Practicing implementing and working with different data structures is essential for mastering programming concepts.
- Familiarity with a variety of data structures equips programmers with the tools needed to tackle a wide range of coding challenges.
- Continued learning and application of data structures will contribute to a programmer's growth and proficiency in software development.
By acknowledging the significance of these key data structures and consistently honing their skills, programmers can enhance their problem-solving abilities and deliver robust solutions in various coding scenarios.